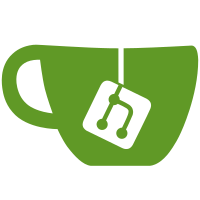
!Breaking change! - The updated version will not be able to read your old database file Major improvements: - Added editable pastas - Added private pastas - Added line numbers - Added support for wide mode (1080p instead of 720p) - Added syntax highlighting support - Added read-only mode - Added built-in help page - Added option to remove logo, change title and footer text Minor improvements: - Improved looks in pure html mode - Removed link to GitHub repo from navbar - Broke up 7km long main.rs file into smaller modules - Moved water.css into a template instead of serving it as an external resource - Made Save button a bit bigger - Updated README.MD Bugfixes: - Fixed a bug where an incorrect animal ID in a request would cause a crash - Fixed a bug where an empty or corrupt JSON database would cause a crash
30 lines
970 B
Rust
30 lines
970 B
Rust
use actix_web::dev::ServiceRequest;
|
|
use actix_web::{error, Error};
|
|
use actix_web_httpauth::extractors::basic::BasicAuth;
|
|
|
|
use crate::args::ARGS;
|
|
|
|
pub async fn auth_validator(
|
|
req: ServiceRequest,
|
|
credentials: BasicAuth,
|
|
) -> Result<ServiceRequest, Error> {
|
|
// check if username matches
|
|
if credentials.user_id().as_ref() == ARGS.auth_username.as_ref().unwrap() {
|
|
return match ARGS.auth_password.as_ref() {
|
|
Some(cred_pass) => match credentials.password() {
|
|
None => Err(error::ErrorBadRequest("Invalid login details.")),
|
|
Some(arg_pass) => {
|
|
if arg_pass == cred_pass {
|
|
Ok(req)
|
|
} else {
|
|
Err(error::ErrorBadRequest("Invalid login details."))
|
|
}
|
|
}
|
|
},
|
|
None => Ok(req),
|
|
};
|
|
} else {
|
|
Err(error::ErrorBadRequest("Invalid login details."))
|
|
}
|
|
}
|