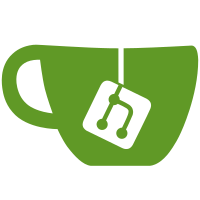
For example, if authentication recovery fails, we load the vault from the database, which may result in a vault format -1 before this commit. We try to avoid this by overwriting the state after the authentication succeeds again, but we can't always avoid the vault format being -1 if, for example, during authentication the user changes activity, so we now store these fields in the database and update them if they have changed during unlock to prevent these states. Alternatively we could have locked the vault but from a UX point of view it makes no sense that the user has to unlock the vault again just because e.g. the token has to be refreshed.
225 lines
6.2 KiB
Groovy
225 lines
6.2 KiB
Groovy
apply plugin: 'org.greenrobot.greendao'
|
|
apply plugin: 'com.android.library'
|
|
apply plugin: 'kotlin-android'
|
|
apply plugin: 'de.mannodermaus.android-junit5'
|
|
|
|
android {
|
|
def globalConfiguration = rootProject.extensions.getByName("ext")
|
|
|
|
compileSdkVersion globalConfiguration["androidCompileSdkVersion"]
|
|
buildToolsVersion globalConfiguration["androidBuildToolsVersion"]
|
|
|
|
defaultConfig {
|
|
minSdkVersion globalConfiguration["androidMinSdkVersion"]
|
|
targetSdkVersion globalConfiguration["androidTargetSdkVersion"]
|
|
|
|
buildConfigField 'int', 'VERSION_CODE', "${globalConfiguration["androidVersionCode"]}"
|
|
buildConfigField "String", "VERSION_NAME", "\"${globalConfiguration["androidVersionName"]}\""
|
|
|
|
testInstrumentationRunner 'androidx.test.runner.AndroidJUnitRunner'
|
|
}
|
|
|
|
compileOptions {
|
|
sourceCompatibility JavaVersion.VERSION_1_8
|
|
targetCompatibility JavaVersion.VERSION_1_8
|
|
|
|
coreLibraryDesugaringEnabled true
|
|
}
|
|
|
|
|
|
buildTypes {
|
|
release {
|
|
buildConfigField "String", "ONEDRIVE_API_KEY", "\"" + getApiKey('ONEDRIVE_API_KEY') + "\""
|
|
buildConfigField "String", "ONEDRIVE_API_REDIRCT_URI", "\"" + getApiKey('ONEDRIVE_API_REDIRCT_URI') + "\""
|
|
}
|
|
|
|
debug {
|
|
buildConfigField "String", "ONEDRIVE_API_KEY", "\"" + getApiKey('ONEDRIVE_API_KEY_DEBUG') + "\""
|
|
buildConfigField "String", "ONEDRIVE_API_REDIRCT_URI", "\"" + getApiKey('ONEDRIVE_API_REDIRCT_URI_DEBUG') + "\""
|
|
}
|
|
}
|
|
|
|
flavorDimensions "version"
|
|
|
|
productFlavors {
|
|
playstore {
|
|
dimension "version"
|
|
}
|
|
|
|
apkstore {
|
|
dimension "version"
|
|
}
|
|
|
|
fdroid {
|
|
dimension "version"
|
|
}
|
|
}
|
|
|
|
sourceSets {
|
|
playstore {
|
|
java.srcDirs = ['src/main/java', 'src/main/java/', 'src/notFoss/java', 'src/notFoss/java/']
|
|
}
|
|
|
|
apkstore {
|
|
java.srcDirs = ['src/main/java', 'src/main/java/', 'src/notFoss/java', 'src/notFoss/java/']
|
|
}
|
|
|
|
fdroid {
|
|
java.srcDirs = ['src/main/java', 'src/main/java/', 'src/foss/java', 'src/foss/java/']
|
|
}
|
|
}
|
|
packagingOptions {
|
|
resources {
|
|
excludes += ['META-INF/DEPENDENCIES', 'META-INF/NOTICE.md', 'META-INF/INDEX.LIST']
|
|
}
|
|
}
|
|
|
|
lint {
|
|
abortOnError false
|
|
ignoreWarnings true
|
|
quiet true
|
|
}
|
|
}
|
|
|
|
greendao {
|
|
schemaVersion 11
|
|
}
|
|
|
|
configurations.all {
|
|
// Check for updates every build (use for cryptolib snapshot)
|
|
//resolutionStrategy.cacheChangingModulesFor 0, 'seconds'
|
|
}
|
|
|
|
dependencies {
|
|
def dependencies = rootProject.ext.dependencies
|
|
|
|
implementation project(':domain')
|
|
implementation project(':util')
|
|
implementation project(':pcloud-sdk-java')
|
|
|
|
coreLibraryDesugaring dependencies.coreDesugaring
|
|
|
|
// cryptomator
|
|
implementation dependencies.cryptolib
|
|
|
|
// greendao
|
|
api dependencies.greenDao
|
|
// dagger
|
|
annotationProcessor dependencies.daggerCompiler
|
|
implementation dependencies.dagger
|
|
|
|
api dependencies.jsonWebTokenApi
|
|
implementation dependencies.jsonWebTokenImpl
|
|
implementation dependencies.jsonWebTokenJson
|
|
|
|
// cloud
|
|
implementation dependencies.dropbox
|
|
implementation dependencies.msgraphAuth
|
|
implementation dependencies.msgraph
|
|
|
|
implementation dependencies.stax
|
|
api dependencies.minIo
|
|
|
|
playstoreImplementation(dependencies.googlePlayServicesAuth) {
|
|
exclude module: 'guava-jdk5'
|
|
exclude module: 'httpclient'
|
|
exclude module: 'googlehttpclient'
|
|
exclude group: "com.google.http-client", module: "google-http-client"
|
|
}
|
|
apkstoreImplementation(dependencies.googlePlayServicesAuth) {
|
|
exclude module: 'guava-jdk5'
|
|
exclude module: 'httpclient'
|
|
exclude module: "google-http-client"
|
|
exclude group: "com.google.http-client", module: "google-http-client"
|
|
}
|
|
|
|
playstoreImplementation(dependencies.googleApiServicesDrive) {
|
|
exclude module: 'guava-jdk5'
|
|
exclude module: 'httpclient'
|
|
exclude module: 'googlehttpclient'
|
|
exclude group: "com.google.http-client", module: "google-http-client"
|
|
}
|
|
apkstoreImplementation(dependencies.googleApiServicesDrive) {
|
|
exclude module: 'guava-jdk5'
|
|
exclude module: 'httpclient'
|
|
exclude module: "google-http-client"
|
|
exclude group: "com.google.http-client", module: "google-http-client"
|
|
}
|
|
|
|
playstoreImplementation(dependencies.googleApiClientAndroid) {
|
|
exclude module: 'guava-jdk5'
|
|
exclude module: 'httpclient'
|
|
exclude module: "google-http-client"
|
|
exclude module: "jetified-google-http-client"
|
|
exclude group: "com.google.http-client", module: "google-http-client"
|
|
exclude group: "com.google.http-client", module: "jetified-google-http-client"
|
|
}
|
|
apkstoreImplementation(dependencies.googleApiClientAndroid) {
|
|
exclude module: 'guava-jdk5'
|
|
exclude module: 'httpclient'
|
|
exclude module: "google-http-client"
|
|
exclude module: "jetified-google-http-client"
|
|
exclude group: "com.google.http-client", module: "google-http-client"
|
|
exclude group: "com.google.http-client", module: "jetified-google-http-client"
|
|
}
|
|
|
|
playstoreImplementation dependencies.trackingFreeGoogleCLient
|
|
apkstoreImplementation dependencies.trackingFreeGoogleCLient
|
|
playstoreImplementation dependencies.trackingFreeGoogleAndroidCLient
|
|
apkstoreImplementation dependencies.trackingFreeGoogleAndroidCLient
|
|
|
|
// rest
|
|
implementation dependencies.rxJava
|
|
implementation dependencies.rxAndroid
|
|
implementation dependencies.okHttp
|
|
implementation dependencies.okHttpDigest
|
|
implementation dependencies.androidAnnotations
|
|
compileOnly dependencies.javaxAnnotation
|
|
implementation dependencies.gson
|
|
|
|
implementation dependencies.commonsCodec
|
|
|
|
implementation dependencies.documentFile
|
|
|
|
implementation dependencies.lruFileCache
|
|
|
|
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk8:$kotlin_version"
|
|
|
|
// test
|
|
testImplementation dependencies.junit
|
|
testImplementation dependencies.junitApi
|
|
testRuntimeOnly dependencies.junitEngine
|
|
testImplementation dependencies.junitParams
|
|
testRuntimeOnly dependencies.junit4Engine
|
|
|
|
testImplementation dependencies.mockito
|
|
testImplementation dependencies.mockitoKotlin
|
|
testImplementation dependencies.mockitoInline
|
|
testImplementation dependencies.hamcrest
|
|
|
|
androidTestImplementation(dependencies.runner) {
|
|
exclude group: 'com.android.support', module: 'support-annotations'
|
|
}
|
|
}
|
|
|
|
configurations {
|
|
all*.exclude group: 'com.google.android', module: 'android'
|
|
}
|
|
|
|
static def getApiKey(key) {
|
|
return System.getenv().getOrDefault(key, "")
|
|
}
|
|
|
|
tasks.withType(Test) {
|
|
testLogging {
|
|
events "failed"
|
|
|
|
showExceptions true
|
|
exceptionFormat "full"
|
|
showCauses true
|
|
showStackTraces true
|
|
|
|
showStandardStreams = false
|
|
}
|
|
}
|